641-design-circular-deque
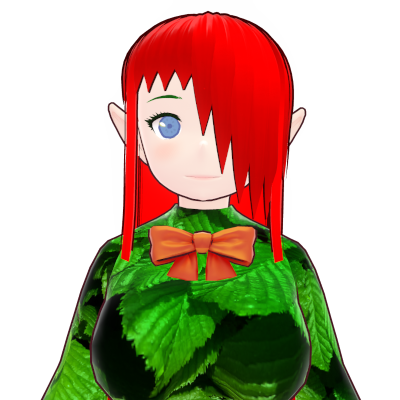
DevGod
Elf Vtuber
/** * @param {number} k */var MyCircularDeque = function(k) { this.data = []; this.maxSize = k; this.currSize = 0;};
/** * @param {number} value * @return {boolean} */MyCircularDeque.prototype.insertFront = function(value) { if(this.currSize < this.maxSize){ this.data.unshift(value); this.currSize++; return true; } return false;};
/** * @param {number} value * @return {boolean} */MyCircularDeque.prototype.insertLast = function(value) { if(this.currSize < this.maxSize){ this.data.push(value); this.currSize++; return true; } return false;};
/** * @return {boolean} */MyCircularDeque.prototype.deleteFront = function() { if(this.currSize > 0){ this.data.shift(); this.currSize--; return true; } return false;};
/** * @return {boolean} */MyCircularDeque.prototype.deleteLast = function() { if(this.currSize > 0){ this.data.pop(); this.currSize--; return true; } return false;};
/** * @return {number} */MyCircularDeque.prototype.getFront = function() { if(this.isEmpty()){ return -1; } return this.data[0];};
/** * @return {number} */MyCircularDeque.prototype.getRear = function() { if(this.isEmpty()){ return -1; } return this.data[this.currSize-1];};
/** * @return {boolean} */MyCircularDeque.prototype.isEmpty = function() { return this.currSize == 0;};
/** * @return {boolean} */MyCircularDeque.prototype.isFull = function() { return this.currSize == this.maxSize;};
/** * Your MyCircularDeque object will be instantiated and called as such: * var obj = new MyCircularDeque(k) * var param_1 = obj.insertFront(value) * var param_2 = obj.insertLast(value) * var param_3 = obj.deleteFront() * var param_4 = obj.deleteLast() * var param_5 = obj.getFront() * var param_6 = obj.getRear() * var param_7 = obj.isEmpty() * var param_8 = obj.isFull() */